Laravel's Most Common Performance Mistakes: How to Avoid Them
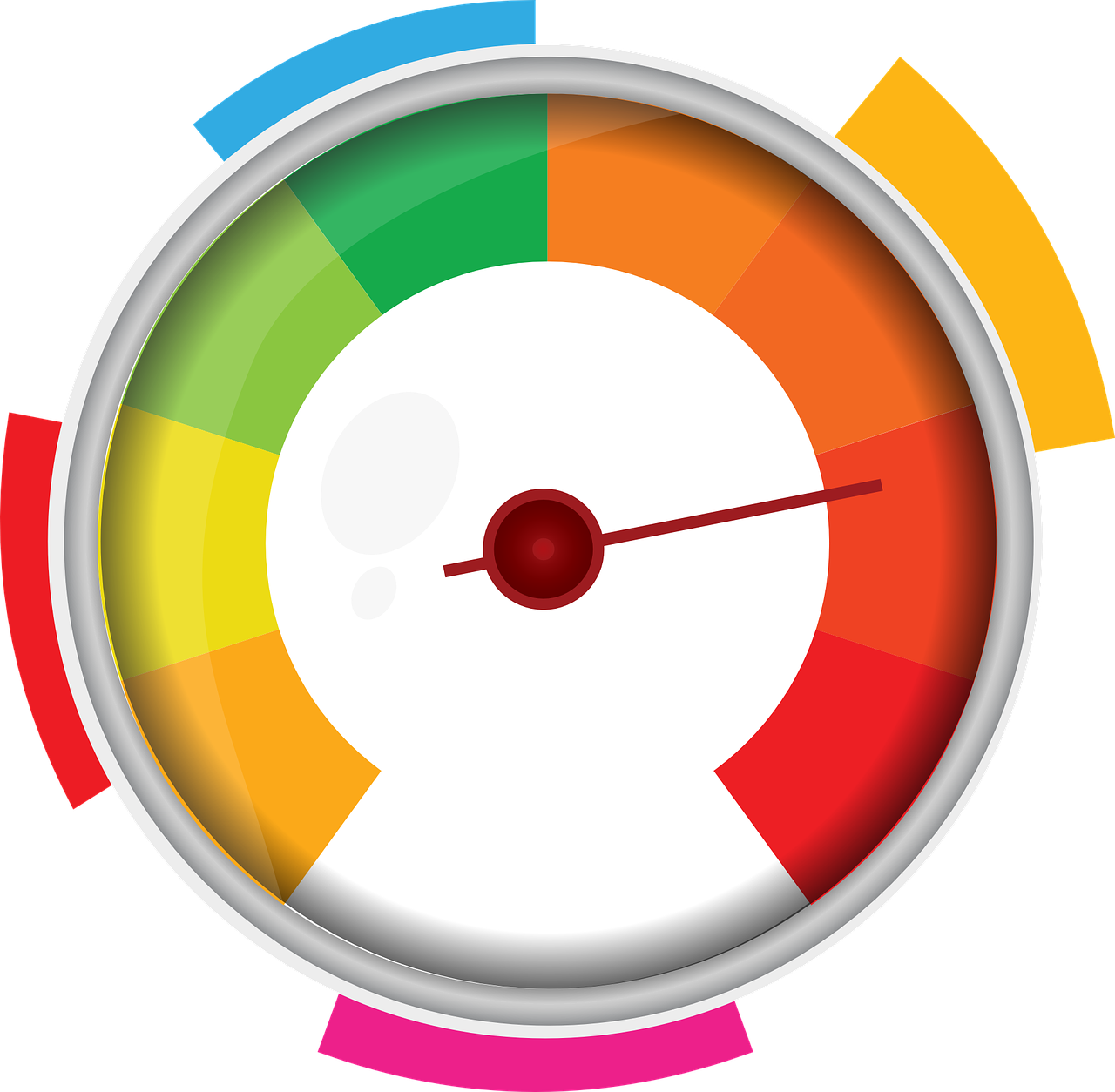
As a Laravel developer, I encounter the same performance issues time and time again. In this article, I'll show you the most common mistakes and how to avoid them.
1. The Famous N+1 Problem
The most common issue of all. Here's a typical example:
// Bad: N+1 Problem $posts = Post::all(); foreach ($posts as $post) { echo $post->author->name; // New query for each post! } // Good: Eager Loading $posts = Post::with('author')->get(); foreach ($posts as $post) { echo $post->author->name; // No additional queries! }
2. Unnecessary Collection Operations
Often collections are used where queries would be better:
// Bad: Loads all users into memory $activeUsers = User::all()->filter(function ($user) { return $user->isActive(); }); // Good: Filters directly in the database $activeUsers = User::where('active', true)->get();
3. Missing Indexes
Missing or incorrect indexes can slow down your application:
// Bad: No index on frequently queried columns Schema::create('orders', function (Blueprint $table) { $table->id(); $table->string('status'); $table->timestamps(); }); // Good: Index on frequently queried columns Schema::create('orders', function (Blueprint $table) { $table->id(); $table->string('status'); $table->timestamps(); $table->index('status'); });
4. Unnecessary Eager Loading
Too much eager loading can be just as bad as too little:
// Bad: Loads unnecessary relationships $posts = Post::with(['author', 'comments', 'tags', 'category', 'media'])->get(); // Good: Loads only needed relationships $posts = Post::with(['author', 'comments'])->get();
5. Poor Cache Strategy
Caching is important, but not always the best solution:
// Bad: Caches data that changes frequently Cache::remember('user_stats', 3600, function () { return User::count(); }); // Good: Caches only slowly changing data Cache::remember('site_settings', 86400, function () { return Setting::all(); });
6. Unoptimized Blade Views
Blade views can cause performance issues:
// Bad: Complex logic in the view @foreach($users as $user) @php $stats = $user->calculateStats(); $permissions = $user->getPermissions(); @endphp {{ $stats['total'] }} - {{ $permissions['admin'] }} @endforeach // Good: Logic in the controller $users = User::with(['stats', 'permissions'])->get();
Best Practices for Better Performance
- Use Laravel's Query Builder efficiently
- Implement caching strategically
- Monitor your database queries
- Use Laravel's Debugbar for performance analysis
- Implement pagination where appropriate
Tools for Performance Analysis
Conclusion
Performance optimization is a continuous process. With these tips, you can avoid the most common mistakes and make your Laravel application faster. Remember: The best performance optimization is the one you don't need to implement.